One of the great ways to reduce the time to review and route a workflow assignment is to setup email notification to inform the users of a new assignment. Some of my clients even want us to provide a more direct, immediate notification on mobile device. This can be done easily (but can be costly) by using a SMS gateway which is a service usually provided by a telecom provider. To use it, you subscribe to a SMS gateway and setup Maximo so that every time you like to inform a user on new assignment, Maximo will sent an email with a predefined format to the gateway. The SMS gateway will send a text message to the user’s mobile phone to inform him/her of the new inbox assignment.
With Push Notification, you can achieve the same result without having to pay a lot of money for SMS gateway service. Apple, Google, Amazon are some of the companies out there which provide a totally free or super cheap Push Notification service.
Thus, in this post, I investigate the possibility of using Google Firebase service to send notification to mobile devices when a person receives new workflow assignment from Maximo.
A – Create a Firebase project on Google Firebase website:
1. Go to https://firebase.google.com, log in using your Google ID, and create a new project. Enter a project name e.g. “Maximo Notification”.
2. After the project has been created, choose “Add Firebase to your Android app”
Enter a package name for the Android Java project that you going to build for receiving Push Notification on Android devices e.g. “io.tqv.maximonotification”. Click Add App
3. After clicked on Add App, the browser will automatically download the “google-services.json” file at step 2 “Copy Config File”- Click Continue. At Step 3: “Add to build.gradle”, quickly skim through the instructions and click Finish.
Sample code of the Android project can be downloaded from this link: GitHub. The steps below are just high level guideline:
2 – Copy the google-services.json file you downloaded in previous step to the project’s app folder.
3 – Add Firebase dependencies to Android project:
Add line: compile ‘com.google.firebase:firebase-messaging:9.6.1’ to app level build.gradle within the dependencies block
Add line: apply plugin: ‘com.google.gms.google-services’ to the bottom of the app level build.gradle
4 – Add a class “MyFirebaseMessagingService.java” which extends FirebaseMessagingService. This will implement the “onMessageReceived” method to handle the message received from Firebase and display Push Notification on the mobile device. You can download sample code of this class here. Make sure to register the service in AndroidManifest.xml by adding the following lines inside Application tag:
5 – To test if the implementation works, register to a hard-coded topic such as: “MAXADMIN” by adding the following line to the onCreate method of the MainActivity.java class:
FirebaseMessaging.getInstance().subscribeToTopic(“MAXADMIN”);
Run the application to register a new topic.
Go to Firebase console, on the left-side menu, click Notifications to open the Notifications page, click New Message to send a new message from Firebase console. Enter some message text, and choose “MAXADMIN” topic for target.
Please note that the first time when a new topic is subscribed, Firebase will automatically add it to the project’s database. However, it will take up to a few hours before a new topic is added and displayed in the “Topic” dropdown of the “New Message” pop-up window, then hit “Send Message”
If the configuration works well, the mobile device will receive and display the notification almost instantly. If it does not show, make a breakpoint at the first line of the onMessageReceived method of the MyFirebaseMessagingService class and send another message to see if the app does receive a message from Firebase or not. If it not, make sure the application have register for INTERNET permission in the AndroidManifest.xml.
6. In the sample code, I added the Preference screen to enable each device to receive notification from one Topic or User. I also added a bit more code to ensure that the service is started when mobile device reboots, and the code to handle the hyperlink data sent within the message so that when the user click on the Notification, it will automatically open the Maximo record in mobile device’s browser for the end-user to review and route workflow.
C – Configure Maximo to send POST request to Firebase:
1. Open Maximo, open Automation Script application, and Select Action to create a new Object Launch Point. Enter a name for launchpoint, the Object should be “WFASSIGNMENT”, with condition “assigncode is not null” (Note: because Maximo use the WFASSIGNMENT table to store both workflow assignment and the configuration Workflow Notes in the Workflow Designer app, this condition ensure that no message is created when someone modify a workflow in the Workflow Designer). The event to trigger the automation script should be “add”. (This means, when a new workflow assignment is created, Maximo will execute this script). Click Next.
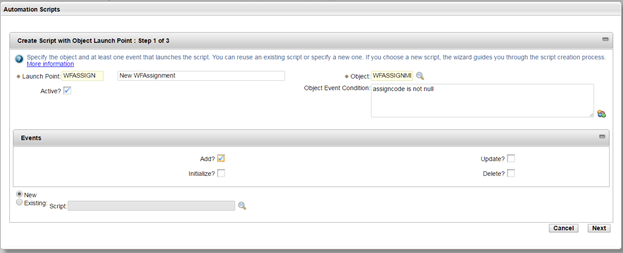
2. In the next page, enter a script name such as “Firebase_Notify”. For script language, choose jython, then click Next.
3. In the next page, copy/paste the sample code into the Source Code text box. And click Create to finish.
This code generates and sends a JSON message via HTTP POST method to Firebase server whenever a new assignment is created. The message also contains a hyperlink to allow the user to open the Maximo record directly when tapping on the Notification. You have to review the code and modify values of the SERVER_KEY and MAX_HOST_NAME constants:
4. To test if the script works well, I created a workflow with only one task node assigned to ‘MAXADMIN’ on the Service Request application. I added the workflow to the Workflow Go button of the Service Request app so that every time I click on the Workflow Route, Maximo will create a new assignment, and trigger the Script to send Firebase a message. I also added a new custom field “errmsg” in the SR object to display the error message of the HTTP response from Firebase in case if it returns an error for easy debugging:
If the message is sent to Firebase successfully, it will send a notification to the mobile device, and when the user tap on the message, it will open the assignment in the device’s browser and allow the user to route workflow to the next step. If you have Maximo Everyplace, it will work very well with the small screen size of smart phone. However, I find that every without Everyplace, it is still possible to review and route workflow without any problem.