Hope you enjoy it.
Category: Service
interface:
- For the getName() function, set a name for the cache, such as “LOCATION_CACHE”.
- Create method loadData() to query and load all locations’
description into a HashSet - Call the loadData() method in the init(), reload(), reload(String)
function overridden from the MaximoCache interface - Create function isLocationExist(String) to check if an
input String matches with a description contained in the HashSet
2. Create a LocationCheck class extending the psdi.server.AppService
class. Override the init() method to initialize a new LocationCache object and
add it to the MXServer’s cache.
4. Extend the LocationSet class to override the fireEventsAfterDBCommit() method. Essentially this method will reload the cache whenever there is a change to location data. This will ensure that the information stored in the cache will always be in sync with the new update.
5. Create a FldDescription class to override the validate()
method, and associate it with the Item.Description field. In the validate()
method, we will check if the description entered is the same as a location’s description stored inside the cache and throw an error dialog if there is a
match.
You can view or download the full sample code from this GitHub repository: link
After deploying the code and associating the LocationSet to
the Location object and the FldDescription class the Description field of the
Item object, to test if the code works, open an Item and enter a description
that matches the description of a location, it should throw an error
message as in the image below:
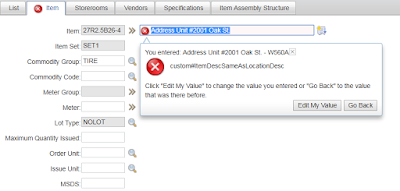
To check if the cache is reloaded properly, create a new location or update an existing one, enter the description of the updated location to an item’s description, it should also throw an error message.